MQL4 How to Avoid Running on Each Tick

Data is continually updated by our staff and systems.
Last updated: 02 Jul 2020
We earn commissions from some affiliate partners at no extra cost to users (partners are listed on our ‘About Us’ page in the ‘Partners’ section). Despite these affiliations, our content remains unbiased and independent. We generate revenue through banner advertising and affiliate partnerships, which do not influence our impartial reviews or content integrity. Our editorial and marketing teams operate independently, ensuring the accuracy and objectivity of our financial insights.
Read more about us ⇾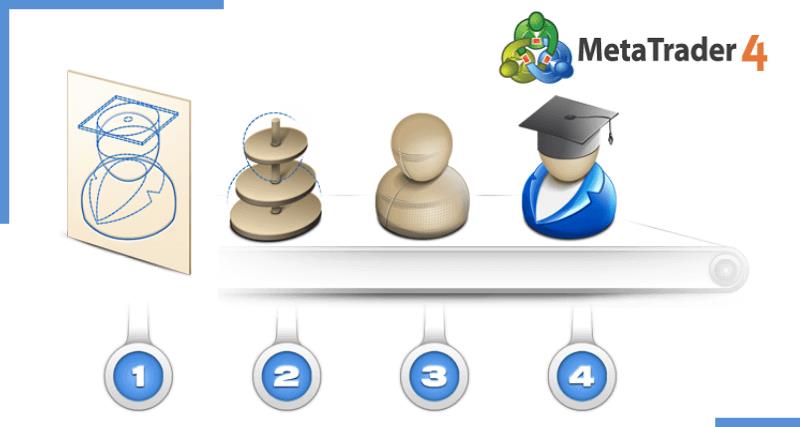
Most expert advisors run in real-time, on every tick, which has its plus side and downside. On the plus side, running on every tick allows the EA to catch the smallest movement in price, and this can be great for a scalping system.
However, on the negative side, executing trades in real-time on every tick can make many systems susceptible to false signals.
Sometimes it is better to check trading conditions only once per bar. By waiting for the bar to close, we can be sure that the condition has occurred and that the signal is valid. Learn in this MQL4 How to Avoid Running on Each Tick article how to program your Expert Advisor to work with bar close price and avoid false signals.
Trading once per bar also means that the results in the Strategy Tester will be more accurate and relevant. Due to the inherent limitations of MT4’s Strategy Tester, using “every tick” as the testing model will produce unreliable back testing results, due to the fact that ticks are often modeled from M1 data. The trades that occur in live trading will not necessarily correspond to trade made in the Strategy Tester.
But by placing our trades on the close of the bar and using “Open prices only” as the testing mode we can get testing results that more accurately reflect real-time trades. The disadvantage of trading once per bar is that trades may be executed late, especially if there is a lot of price movement over the course of the bar. It’s basically a trade-off between responsiveness and reliability.
There are two ways that I know that can check the trade conditions once per bar: 1) time stamp method; and 2) volume method;
1. Time Stamp Method
Here is the code for the time stamp method to check for the opening of the new bar.
int CurrentTime;
int init(){
CurrentTime= Time[0];
return(0);
}
int start(){
if (EnterOpenBar) = true)
{
if(CurrentTime != Time[0]){
// first tick of new bar found
// buy and sell conditions here
CurrentTime= Time[0];
return(0);
}
}
Here in this method, we first declare an external variable named EnterOpenBar to turn the feature on and off.
In the init() function we will assign the time stamp of the current bar to CurrentTime. This will delay the trade condition check until the opening of the next bar.
Next we compare the value of the CurrentTime variable to Time[0], which is the time stamp of the current bar. If the two values do not match, then a new bar is detected and we can proceed to open up trade on new bar.
2. Volume Method
Here is the code for the volume method for checking the opening of new bar.
int start ()
bool OpenBar=true;
if(EnterOpenBar) if(iVolume(NULL,0,0)>1) OpenBar=false;
if (OpenBar) {
// first tick of new bar found
// buy and sell conditions here
return (0);
}
I prefer the volume method because it contains much shorter pieces of code.
As in the previous method, we first declare an external variable named EnterOpenBar to turn the feature on and off. We do not need anything in the init() function.
We just need to put in that one line of code to check for the openbar condition based on volume. This condition checks to see if the volume is 1, in which case OpenBar=true because it has found the first tick of the new bar. If the volume is greater than 1 (>1) OpenBar = false because it is no longer the new bar.